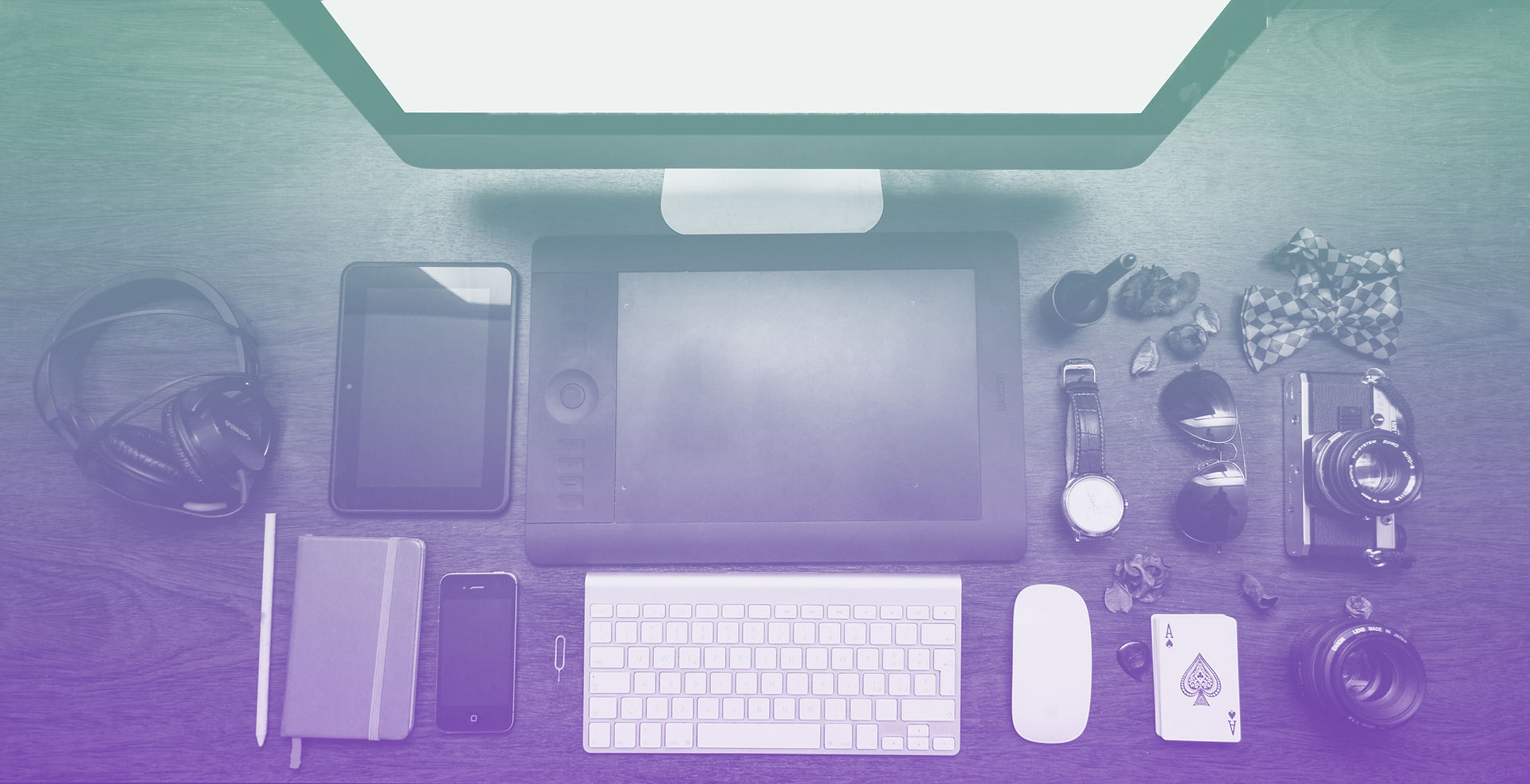
PHP
PHP è un linguaggio di scripting, ed è un potente strumento per rendere le pagine Web dinamiche e interattive.
Sintassi
<?php
istruzione php
?>
Variabili
$nomeVariabile="ciao";
$nomeVariabile= 23;
Tipi di variabili
-
String:
concatenazione di string
$stringa = $s.$p.$"ciao"; -
Integer
-
Float (floating point numbers - also called double)
-
Boolean
-
Array
-
Object
-
NULL
-
Resource
ECHO o PRINT
Per stampare qualcosa
<?php
echo "<h2>PHP is Fun!</h2>";
echo "Hello world!<br>";
echo "I'm about to learn PHP!<br>";
echo "This ", "string ", "was ", "made ", "with multiple parameters.";
?>
<?php
print "<h2>PHP is Fun!</h2>";
print "Hello world!<br>";
print "I'm about to learn PHP!";
?>
OPERATORI
+ (addizione) => $x + $y
- (sottrazione) => $x - $y
* (moltiplicazione) => $x * $y
/ (divisione) => $x / $y
%(modulo) => $x % $y
= (assegnazione) => $x=$y
== (uguale) => $x==$y
< (maggiore) => $x>$y
> (minore) => $x<$y
!=(diverso) => $x!=$y
|| (Or) => $x==$y || $x>$z
&& (And) => $x==$y && $x>$z
! (not) => !$x
. (concatenazione) => $txt1.$txt2
.= (concatenazione all'assegnamento) => $txt1.=$txt2
Istruzioni di condizione
Istruzione if; if...else...; if..elseif..else
if (condizione)
{
istruzione
//codice da eseguire se condizione è vera
}
else
{ istruzione
// codice da eseguire se istruzione è falsa
}
esempio1
<?php
$t = date("H");
if ($t < "20") {
echo "Have a good day!";
}
?>
esempio2
<?php
$t = date("H");
if ($t < "20") {
echo "Have a good day!";
} else {
echo "Have a good night!";
}
?>
if (condizione) {
codice da eseguire se condizione è vera;
} elseif (condizione) {
codice da eseguire se condizione è vera;
} else {
codice da eseguire se condizione è falsa;
}
esempio
<?php
$t = date("H");
if ($t < "10") {
echo "Have a good morning!";
} elseif ($t < "20") {
echo "Have a good day!";
} else {
echo "Have a good night!";
}
?>
Istruzione switch
switch (n) {
case label1:
codice da eseguire se if n=label1;
break;
case label2:
codice da eseguire se if n=label2;
break;
case label3:
codice da eseguire se if n=label3;
break;
...
default:
codice da eseguire se n è differente da tutti i label;
}
esempio
<?php
$favcolor = "red";
switch ($favcolor) {
case "red":
echo "Your favorite color is red!";
break;
case "blue":
echo "Your favorite color is blue!";
break;
case "green":
echo "Your favorite color is green!";
break;
default:
echo "Your favorite color is neither red, blue, or green!";
}
?>
while loop
while (condizione è vero) {
codice da eseguire;
}
esempio
<?php
$x = 1;
while($x <= 5) {
echo "The number is: $x <br>";
$x++;
}
?>
do {
codice da eseguire;
} while (condizione è vero);
esempio
<?php
$x = 1;
do {
echo "The number is: $x <br>";
$x++;
} while ($x <= 5);
?>
for loop
for (inizializza contatore; verifica contatore; incrementa contatore) {
codice da eseguire
}
esempio
<?php
for ($x = 0; $x <= 10; $x++) {
echo "The number is: $x <br>";
}
?>
PHP function
function nomeFunzione() {
codice da eseguire;
}
esempio
<?php
function writeMsg() {
echo "Hello world!";
}
writeMsg(); // call the function
?>
Array
funzione per creare un array: array();
assegnazione automatica
$cars = array("Volvo", "BMW", "Toyota");
assegnazione manuale
$cars[0] = "Volvo";
$cars[1] = "BMW";
$cars[2] = "Toyota";
Per ottenere la lunghezza del vettore
<?php
$cars = array("Volvo", "BMW", "Toyota");
echo count($cars);
?>
Array associativa
$age = array("Peter"=>"35", "Ben"=>"37", "Joe"=>"43");
or
$age['Peter'] = "35";
$age['Ben'] = "37";
$age['Joe'] = "43";
PHP date
La funzione del PHP date/time è una parte del PHP CORE. Perciò non richiede un ulteriore installazione per utilizzo.
Alcuni funzioni principali
-
date_create("year-month-day");
Restituisce la data oppure "false" al fallimento della funzione.
prova
-
date_format(date, format);
Restituisce la data nel formato indicato.
Esempio:
<? php
date_create_from_format("J-M-Y", "15-mar-2013");
echo date_format($date, "y/m/d");
?>
-
date_create_from_format(format,time,timezone);
Restituisce un nuovo formato della data.
Formato:
d - Day of the month; with leading zeros
j - Day of the month; without leading zeros
D - Day of the month (Mon - Sun)
l - Day of the month (Monday - Sunday)
S - English suffix for day of the month (st, nd, rd, th)
F - Monthname (January - December)
M - Monthname (Jan-Dec)
m - Month (01-12)
n - Month (1-12)
Y - Year (e.g 2013)
y - Year (e.g 13)
a and A - am or pm
g - 12 hour format without leading zeros
G - 24 hour format without leading zeros
h - 12 hour format with leading zeros
H - 24 hour format with leading zeros
i - Minutes with leading zeros
s - Seconds with leading zeros
u - Microseconds (up to six digits)
e, O, P and T - Timezone identifier
U - Seconds since Unix Epoch
(space)
# - One of the following separation symbol: ;,:,/,.,,,-,(,)
? - A random byte
* - Rondom bytes until next separator/digit
! - Resets all fields to Unix Epoch
| - Resets all fields to Unix Epoch if they have not been parsed yet
+ - If present, trailing data in the string will cause a warning, not an error
-
checkdate(month, day, year);
Month = può essere un numero compreso tra 1 e 12;
Day = può essere un numero compreso tra 1 e 31;
Year = può essere un numero compreso tra 1 e 32767;
Restituisce TRUE se la data è valida altrimenti restituisce il FALSE .
-
date_add(object, interval);
Aggiunge un intervallo alla data.
Esempio:
<? php
$date = date_create("2013-03-15");
date_add($date, date_interval_create_from_date_string("40 days"));
echo date_format ($date, "y-m-d");
?>
-
date_diff(datetime1, datetime2, absolute);
restituisce la differenza tra due date.
Esempio:
<? php
$date1 = date_create("2013-03-15");
$date2 = date_create("2013-12-12");
$diff= date_diff($date1, $date2);
echo diff;
?>
-
date_modify(object, modify);
Serve a modificare una data.
Esempio:
<?php
$date = date_create("2013-05-01");
date_modify($date, "+15days");
echo date_format($date, "y-m.d");
?>
-
date_sub(object, intervallo);
Toglie un intervallo alla data.
Esempio:
<? php
$date = date_create("2013-03-15");
date_sub($date, date_interval_create_from_date_string("40 days"));
echo date_format ($date, "y-m-d");
?>
restituisce: 2013-02-03
PHP MySQL
connessione al DB
$conn = mysql_connect("localhost", "root", "") or
die('Errore di connessione: ' . mysql_error());
selezione del DB
$db_selected = mysql_select_db("quiz patente b", $conn) or
die ('Errore selezione DB: ' . mysql_error());
eseguire una query
$query="SELECT * FROM quiz";
$result = mysql_query($query);
//controllo se è andato a buon fine
if (!$result) {
$message = ' query non valida: ' . mysql_error() . "\n";
$message .= 'Testo query: ' . $query;
die($message); }
per ottenere il numero totale di righe del risultato della query
$num_rows = mysql_num_rows($result);
se il risultato è uno
$row=mysql_fetch_array($result);
$variabile=$row['nomecampo'];
se il risultato è maggiore di uno
while($row = mysql_fetch_assoc($result)) {
$var1=$row['nomecampo1'];
$var2=$row[nomecampo2'];
ecc....